C is one of the most popular and widely used programming languages in the world. It is a low-level language that gives programmers direct access to the hardware and memory. C is also the basis for many other languages such as C++, Java, Python and PHP. Learning C can help you understand the fundamentals of programming and improve your problem-solving skills.
However, C is not an easy language to master. It has many features and rules that can be confusing or tricky for beginners and even experienced programmers. One of the best ways to learn C is to practice writing and reading code. By doing so, you can familiarize yourself with the syntax, the structure and the logic of C programs.
In this article, we will present you with four coding questions on C program output. These questions will test your knowledge of C concepts such as variables, pointers, arithmetic operators and swapping. We will also provide you with the solutions and explanations for each question. By the end of this article, you will have a better understanding of how C programs work and how to write efficient and correct C code. Let’s get started!
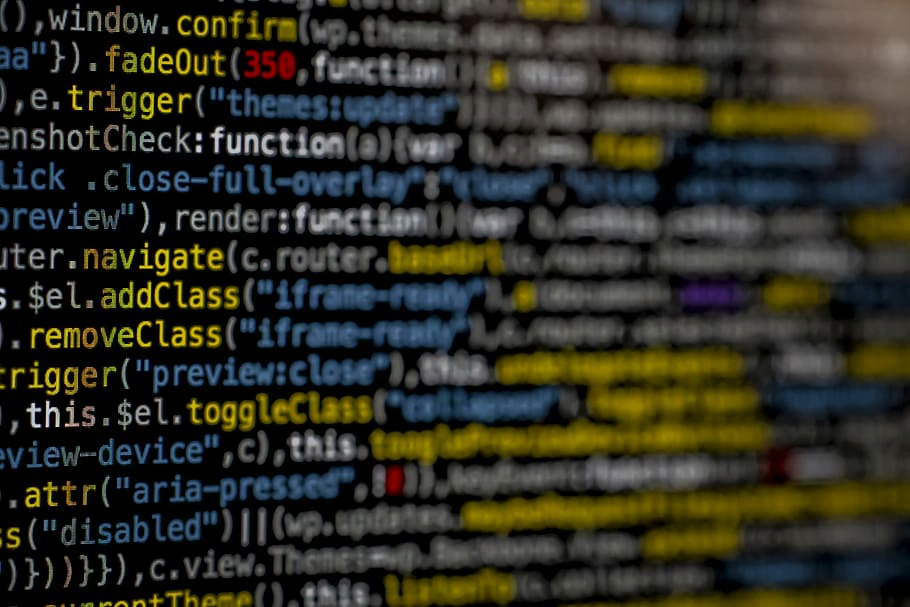
Contents
1st Example – The Output of the Following C Code
Understanding the output of a piece of code is crucial for any programmer. In the case of the C programming language, where precision and predictability are paramount, dissecting the output is a skill every coder must master. Let’s delve into a specific C code snippet and analyze what it will produce.
The C Code
#include <stdio.h>
int main() {
int x = 10, y = 20, z = 30;
int *ptr;
ptr = &x;
printf("%d ", *ptr);
ptr = &y;
printf("%d ", *ptr);
ptr = &z;
printf("%d ", *ptr);
return 0;
}
C Code Analysis
In this code snippet, we declare three integer variables: x
, y
and z
with values 10
, 20
and 30
respectively. Additionally, we declare a pointer variable ptr
of type integer.
ptr = &x;
assigns the address ofx
to the pointerptr
.printf("%d ", *ptr);
prints the value pointed to byptr
which is10
.ptr = &y;
now pointsptr
to the address ofy
.printf("%d ", *ptr);
prints the value pointed to byptr
which is20
.ptr = &z;
finally,ptr
is directed to the address ofz
.printf("%d ", *ptr);
prints the value pointed to byptr
which is30
.
C Code Output
When this code is executed, the output will be:
10 20 30
2nd Example – Understanding C Code Outputs
This example demonstrates the basic arithmetic operators in C and highlights the importance of understanding the nuances of integer division and remainders.
#include <stdio.h>
int main() {
int a = 5;
int b = 2;
printf("a + b = %d\n", a + b);
printf("a - b = %d\n", a - b);
printf("a * b = %d\n", a * b);
printf("a / b = %d\n", a / b);
printf("a %% b = %d\n", a % b);
return 0;
}
Breaking Down the Code:
- Header File Inclusion: The code includes the <stdio.h> header file, which provides essential input-output functions such as printf.
- Main Function: The main function is the entry point of the program. It defines variables and performs operations.
- Variables: Two integer variables, a and b, are declared. a is initialized with the value 5, and b is initialized with the value 2.
- printf Statements: Five printf statements are used to print the results of various mathematical operations on a and b.
Analyzing the Output:
- Addition: The a + b expression evaluates to 5 + 2, resulting in an output of 7.
- Subtraction: The a – b expression evaluates to 5 – 2, resulting in an output of 3.
- Multiplication: The a * b expression evaluates to 5 * 2, resulting in an output of 10.
- Division: The a / b expression performs integer division, which means it discards the fractional part. 5 / 2 evaluates to 2.
- Remainder: The a % b expression calculates the remainder after dividing 5 by 2, which is 1.
Running this code will produce the following output:
a + b = 7
a - b = 3
a * b = 10
a / b = 2
a % b = 1
3rd Example – Decoding the Output of C Code Snippets
#include <stdio.h>
int main() {
int a = 5, b = 10, c = 0;
c = a++ + ++b;
printf("a = %d, b = %d, c = %d\n", a, b, c);
return 0;
}
To understand the output of this code, let’s break down each line and examine its purpose.
#include <stdio.h>
This line includes the standard input/output library, which provides functions like printf() for displaying output and scanf() for reading input from the user.
int main()
This line declares the main() function, which is the entry point of a C program. All C programs must have a main() function, and it is where the program execution begins.
int a = 5, b = 10, c = 0;
In this line, we declare three integer variables: a, b and c. The values 5, 10 and 0 are assigned to a, b, and c respectively.
c = a++ + ++b;
This line is where the magic happens. Let's break it down:
a++
This is a post-increment operation. The value of a (which is currently 5) is first used for the calculation, and then a is incremented by 1 after the calculation. So, the value used in the calculation is 5, and after the calculation, a becomes 6.
++b
This is a pre-increment operation. Before the calculation, the value of b is incremented by 1. So, the value of b becomes 11 before it is used in the calculation.
a++ + ++b
The calculation is performed as follows: 5 + 11 = 16. The value of c is assigned the result, which is 16.
printf("a = %d, b = %d, c = %d\n", a, b, c);
This line uses the printf() function to display the final values of a, b, and c. The %d placeholders are replaced with the values of the variables in the order they appear (a, b, and c) and the newline character \n ensures that a new line is printed after the output.
return 0;
This line indicates that the program has finished executing successfully and it returns a value of 0 to the operating system.
When we run this code, the output will be:
a = 6, b = 11, c = 16
4th Example – Unraveling the Output from C Code Samples
The provided C code is a classic example of swapping the values of two variables without using a temporary variable. Here’s the code:
#include<stdio.h>
int main()
{
int x = 10, y = 20;
int *p, *q;
p = &x;
q = &y;
*p = *p + *q;
*q = *p - *q;
*p = *p - *q;
printf("x = %d, y = %d\n", x, y);
return 0;
}
Let’s break down how this works:
- Point 1 – Two integer variables x and y are declared and assigned the values 10 and 20 respectively.
- Point 2 – Two pointer variables p and q are declared and assigned the addresses of x and y respectively. This means that p points to x and q points to y.
- Point 3 – The following three statements are used to swap the values of x and y:
*p = *p + *q;
This adds the values of x and y and stores the result in x. Now x becomes 30 and y remains 20.*q = *p - *q;
This subtracts the value of y from x and stores the result in y. Now x remains 30 and y becomes 10.*p = *p - *q;
This subtracts the value of y from x and stores the result in x. Now x becomes 20 and y remains 10.- Finally, the printf function is used to print the values of x and y. The %d format specifier is used to print integers and the \n escape sequence is used to print a new line.
The output of this code will be:
x = 20, y = 10
Conclusion
These coding questions on C program output are both challenging and enlightening. They can help you sharpen your coding skills and prepare for coding tests and interviews by showing you how to interpret code.
Let us know what you think about these questions in the comments and follow us for more coding quizzes. To write effective and correct C code, you need to know how a C program works. By exploring code examples like this, you can learn more about the syntax and logic of C language and enhance your coding abilities.
To determine the output of a C program, you need to pay attention to the syntax, the structure and the reasoning of the code.
This example shows how to declare and use pointer variables in C. By breaking down the code into smaller parts, we can easily understand how the code works and what it produces.
This method can help us understand and debug more complex C programs. Happy coding!